What Is an ORM and How Does It Work?
Nov 2024
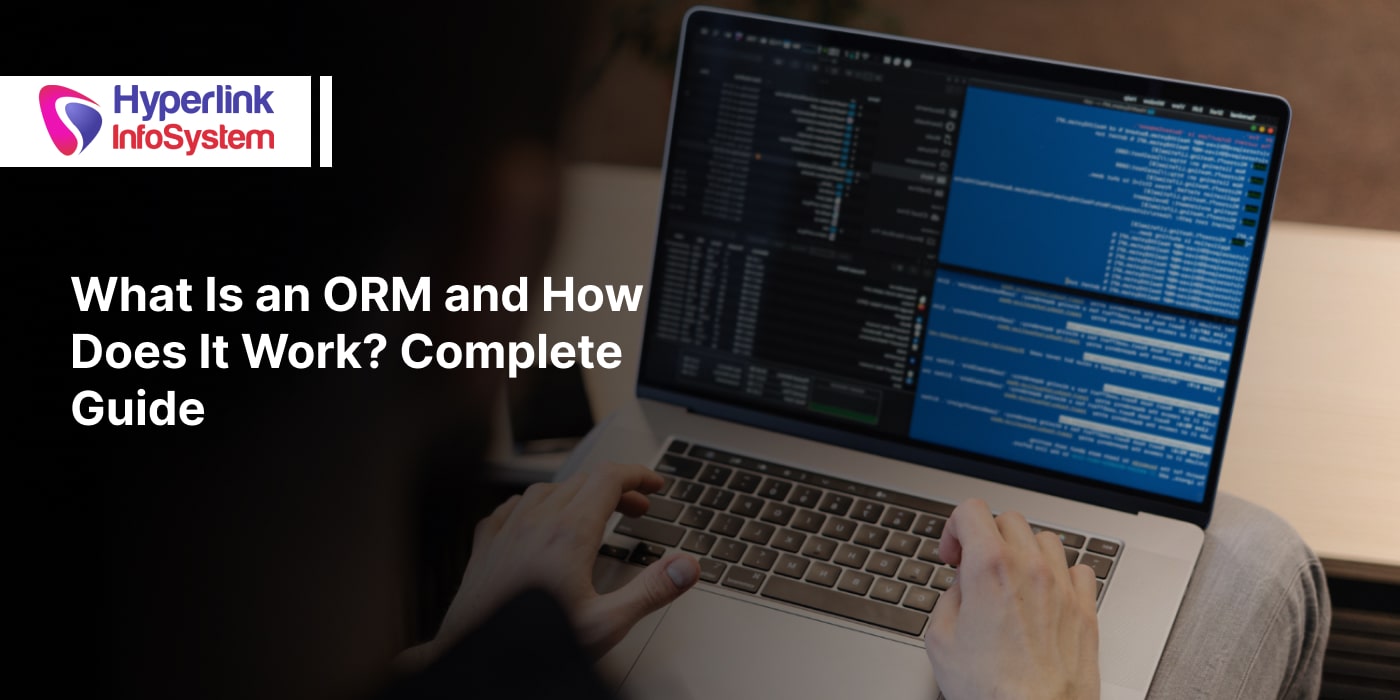
Effective data management is the major concern of the developers in the current software development. The most effective technologies for streamlining interactions with a database are ORM (object-relational mapping) technologies. Because they make easy transfer of data between relational databases and object-oriented programming languages, ORMs have become an integral part of the software development life cycle. It is an ORM framework that simplifies data administration and raises productivity because the developers do not have to write complex SQL queries.
In this blog, we'll discuss the definition, working, and importance of ORMs in today's tech stacks of software development. Afterward, you can work more effectively and efficiently on your project, whether you are a software house or trying to hire software developers.
What Is ORM?
A programming approach named object-relational mapping (ORM), removes the overhead of SQL codes; developers instead work with relational databases using object-oriented programming languages. For this reason, ORM tools play the role of an interface between the data in a database and objects in code, thus enabling developers to edit data records as if they were common programming objects. This accelerates and minimizes the chances of errors in the development process by making the retrieving, inserting, and modification processes for data easier.
ORMs are the most vital components in software development because they make database operations easier, and that programs work with data properly, even consistently. Among the best and most known tech stacks that constitute software development, several make use of the ORM frameworks - Hibernate, Django ORM, and Entity Framework, among others.
How Do ORM Tools Work?
The way most ORM tools work is by mapping the objects in your code to tables in a relational database so that database records may appear to be standard objects in the programming language. Behind the scenes, the ORM framework automatically produces and runs all of the SQL queries required to add, read, edit, and remove objects in an application.
Having discussed above, the operations of ORM tools while developing software are segmented as below.
- ORM tools map object-oriented classes to database tables and their characteristics to columns in the table. For instance, the "users" table in the database may match with a "User" class in code.
Here’s an example using Python's SQLAlchemy ORM:
from sqlalchemy import Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
email = Column(String)
- Operations on Data Using Objects: Developers do not write unformatted SQL statements; instead, they work directly with objects. The developer only needs to make queries on an object model; the ORM will then translate that action into a similar SQL query. For instance, when you query a list of users, the ORM handles converting that action into a corresponding SQL query:
# Querying the database using ORM
users = session.query(User).all()
- Automatic SQL Generation: With the ORM tool, one does not need to write SQL code as it automatically creates the SQL statements to insert, update, or delete records in the database whenever a developer adds or edits objects.
# Adding a new user
new_user = User(name='John Doe', email='john@example.com')
session.add(new_user)
session.commit()
# The ORM automatically generates:
# INSERT INTO users (name, email) VALUES ('John Doe', 'john@example.com')
ORMs have become an integral component of modern software development tech stacks due to their automated approach that makes data administration even simpler in complicated applications. It helps software development companies deal with large-scale data activities efficiently, maintain cleaner code that is more understandable, and develop at a higher speed.
List of Popular ORM Tools
To simplify database interaction, in software development, many ORM tools are in general use. Among the most widely used ORM frameworks, which are nowadays often considered indispensable in many tech stacks for software development, are the following:
1) Hibernate in Java
These are the kinds of frameworks that are valued for the features they offer: Hibernate is the most popular ORM framework for Java and supports complex features such as caching, slow loading, and automatic schema building.
2) C#/.NET Entity Framework
For .NET developers, Microsoft's Entity Framework is the most common ORM solution because it supports strong LINQ data access and promotes the opportunity to interact with databases without SQL scripting.
3) Python Django ORM
This ORM system is part of the Django framework and allows using relational databases in an easier manner in Python. It is excellent for web development-related tasks and supports a lot of databases.
4) The Python SQLAlchemy
Another very powerful ORM, SQLAlchemy allows developers to switch between high-level ORM patterns or even lower-level SQL code whenever needed while performing complex database schemas.
5) Ruby's Rails Active Record
Active Record is the default ORM for Ruby on Rails. It turns database tables into Ruby classes with which database operations become nearly as easy as object-oriented work. This makes it perfect for rapidly building applications for the web.
Benefits of Using ORM Tools
- Simplified Database Management
The removal of complexity from SQL queries, along with the process of interaction with the database, facilitates developers to use databases through widely-known object-oriented programming techniques through ORM technologies.
- Faster Development
Developers working on using ORMs do not have to waste their valuable time writing repetitive SQL code; rather, they can focus on the business logic, and this allows teams to complete the projects faster and shorten the software development life cycle.
- Better Maintainability of Codes
ORM frameworks eliminate redundancy and, therefore, encourage cleaner, more structured code, which makes it much easier to maintain and upgrade an application over time.
- Cross-Database Compatibility
Most ORMs support multiple database systems, which makes an application more flexible and future-proof by allowing developers the ability to change databases with little alteration to code.
- Better Security
While ORMs automatically maintain SQL query construction and clean inputs, they improve the overall security of your software development project and prevent SQL injection attacks.
- Reduction of Boilerplate Code
ORMs strongly reduce boilerplate code as database operations such as inserts, updates, and deletions are automated, making the codebase easier to understand and comprehend.
- Easier Cooperation
ORMs make it easier for committed groups of software engineers to work together with the abstraction of complicated SQL and offer a standard, understandable method of data management.
Any software development organization wishing to strengthen long-term maintainability, increase efficiency, and streamline development processes would do well to invest in ORM frameworks.
Cons of Using ORM Tools
- Overhead of Performance
Because the abstraction layer will get less efficient SQL compared to hand-written queries, ORM tools bring in the cause of performance problems, especially with sophisticated questions. This leads to database operations getting slowed down.
- Lack of Control Over Queries
At times, developers lose fine-grained control over SQL queries by involving intricate and specialized queries that require specific performance tuning or optimizations.
- Learning Curve
While ORM frameworks make the interaction with databases relatively easier, there is not a special "pass to paradise" for it either. There is, of course, some effort that must be put in first to learn and understand its inner mechanisms and conventions.
- Overhead in Use Cases
A basic ORM is too much overhead for small programs without vast database needs, and in places where simple SQL would be sufficient, it introduces unnecessary complexity and resource overheads.
- Obscured Complexity
ORM solutions, especially for newer developers, tend to hide the subtleties of database interactions that may often result in poorly optimized queries or a poor understanding of data management.
- Migration Issues
Theoretically, switching databases with an ORM is a piece of cake; in reality, compatibility issues and schema migration across multiple database systems, especially with database-specific features, can come into play.
Nonetheless, many software development companies still prefer ORM because of its productivity and ease of use, especially with competent programmers who can find a way around any such complexity or performance issues.
ORM vs SQL: Comparison
SQL and Object-Relational Mapping (ORM) tools are the essentials of working with databases in software development but are significantly different in use and method. Orms allow programmers to work through their application objects with object-oriented code, automatically converting the data between those objects and their corresponding database tables. This negates much of the necessity to hand-craft complex SQL queries, improves productivity, and makes database development easier. ORMs are therefore extremely useful on large projects where there is a need to maintain code bases and develop fast.
However, SQL is directly much more powerful as far as the control of the database is concerned, hence enabling developers to make specific queries that may be unique and tailored for certain purposes. Although SQL has better efficiency in some cases, mainly when some complicated queries are used, it requires a better understanding of database administration, thus increasing the possibility of code duplication. The decision to use ORM over SQL, or vice versa, is often determined by the project's complexity and the requirement for fine-grained query optimization; larger and more complex applications tend towards the use of an ORM framework, and performance and control are more crucial when raw SQL is used.
Conclusion
To put it simply, ORM technologies present an easier process in database management in software development so developers should concentrate more on business logic than complex SQL queries. While ORMs make code maintenance easier and increase productivity, they also possess a couple of performance and control trade-offs. Ultimately, it is all about the needs and scope of your project between the application of ORM and SQL.
With the best ORM frameworks, our experienced software developers will be able to help you build scalable and reliable apps. Hyperlink Infosystem is a leading software development company that provides a solution uniquely tailored to your needs. Contact us today to hire dedicated developers and push your project ahead!
Frequently Asked Questions
Depending on the implementation, ORM can be either a library or a framework. It facilitates database interactions in software development by offering a set of tools for the mapping of objects in code to relational database tables.
Whereas an ORM handles the mapping of the object-oriented code as well as the database tables, permitting easy execution of database operations without, of course, the typing of SQL queries, an API refers to communication between multiple software components.
Hibernate (Java), Entity Framework (C#), Django ORM (Python), SQLAlchemy (Python), and Sequelize for Node.js.
ORM tools are much safer in the idea since security risks from database interactions through them are minimized by automatically taking care of sanitizing inputs and formulating queries, which increases the possibility of preventing SQL injection attacks.
Latest Blogs
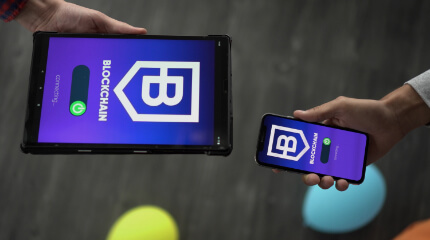
Is BlockChain Technology Worth The H ...
Unfolds The Revolutionary & Versatility Of Blockchain Technology ...
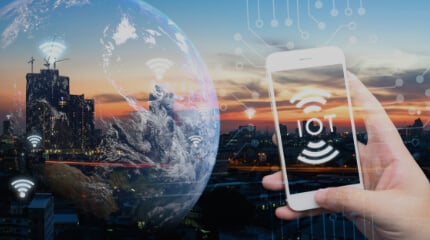
IoT Technology - A Future In Making ...
Everything You Need To Know About IoT Technology ...
Feel Free to Contact Us!
We would be happy to hear from you, please fill in the form below or mail us your requirements on info@hyperlinkinfosystem.com
Hyperlink InfoSystem Bring Transformation For Global Businesses
Starting from listening to your business problems to delivering accurate solutions; we make sure to follow industry-specific standards and combine them with our technical knowledge, development expertise, and extensive research.
4500+
Apps Developed
1200+
Developers
2200+
Websites Designed
140+
Games Developed
120+
AI & IoT Solutions
2700+
Happy Clients
120+
Salesforce Solutions
40+
Data Science