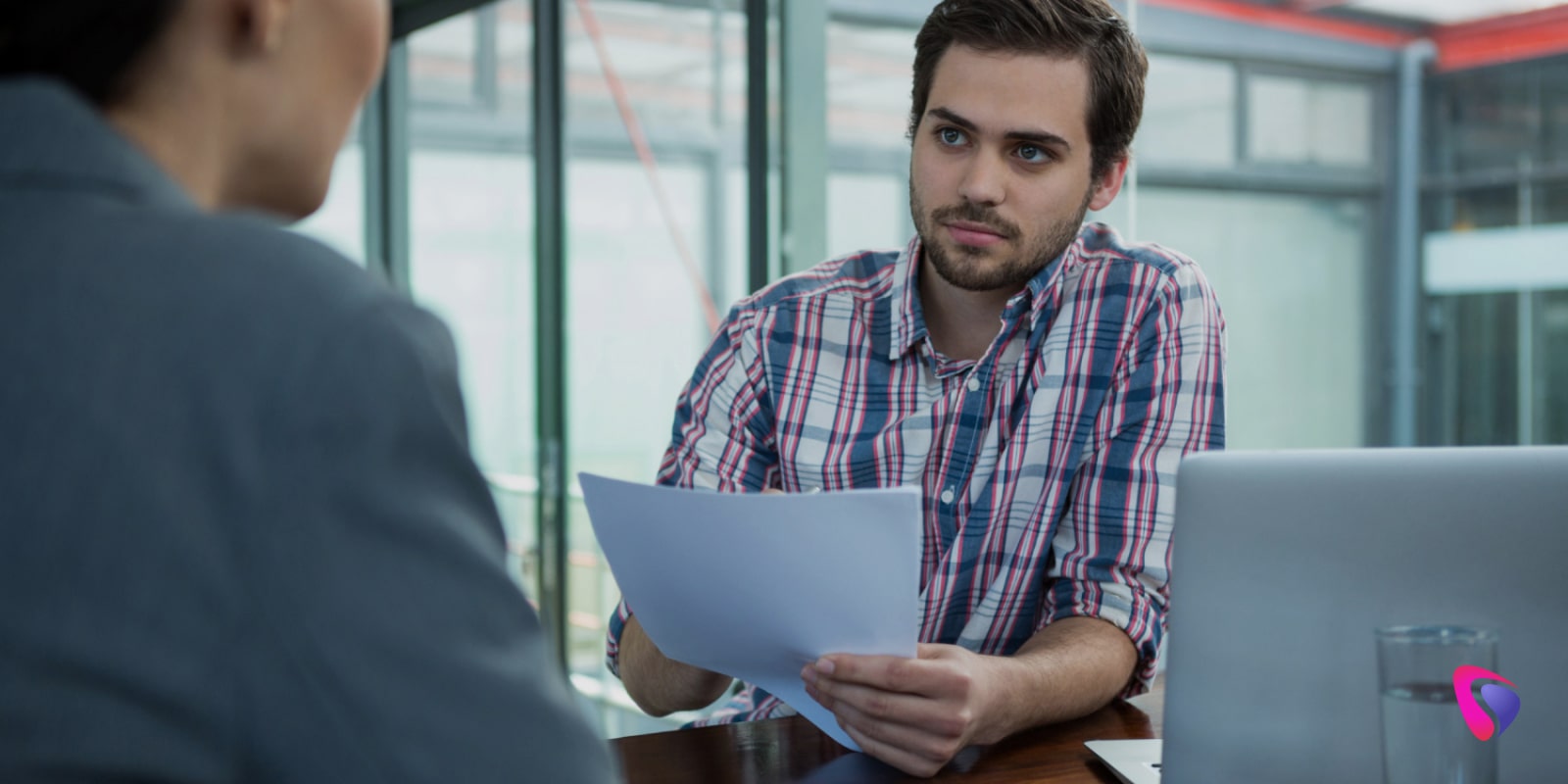
To crack the interview in this furious competition of mobile app development, one needs to have a good hold over both core concepts and cutting-edge methods unique to Android as well as iOS. This article sums up the list of frequently asked interview questions ranging from very basic subjects such as intents and multithreading to relatively complex ideas such as dependency injection, architectural patterns, and framework-specific tools. All these questions and answers would help you prepare more confidently and successfully for your next interview, whether you have a little experience or a lots as a developer.
Mobile App Developer Interview Questions/Answers
Q1. How do you ensure the performance and responsiveness of a mobile app?
Ans. I make use of such tools as AsyncTask or ExecutorService (in Android) or Coroutines (for Kotlin) to make sure that a mobile application is delivered from heavy operations such as network calls and processing on the main UI thread to ensure its performance and responsiveness. As an additional resource, I use profiling tools such as Instruments for iOS and Android Profiler to monitor resources with a view to optimization of memory usage in the application. And to optimize network queries, I also use lazy loading strategies and cache methods for the sake of accelerating the app load speeds. Frequent testing of the app across a range of devices and performance levels can help ensure that it continues to function responsively under varied conditions.
Q2. Describe your experience with cross-platform mobile app development frameworks.
Ans. Here, you can explain your experience with cross-platform frameworks you've worked on. Be honest about your experience. For example:
I have worked on cross-platform frameworks such as Xamarin, React Native, and Flutter for years. That's been saving time and effort on development because it allows running a single codebase on iOS and Android. For instance, Flutter provides lots of pre-made widgets with native-like performance and uses the Dart programming language. The working JavaScript-based React Native can be applied to apps that demand highly interactive user interfaces and real-time changes. C# is applied in Xamarin to maintain native performance with cross-platform code sharing. These frameworks have proven handy in producing cross-platform, quick solutions that do not compromise on quality.
Q3. How to manage state in a mobile application?
Ans. Usually, I would employ platform-specific techniques when it comes to state control within mobile applications. For Android, I use architecture components such as ViewModel and LiveData. In this kind of setup, the ViewModel saves UI-related data in lifecycle-aware fashion, so it survives configuration changes, like screen rotations. There's no need to think about memory leaks due to LiveData; it just makes it easy to watch the changes of data and immediately update the UI in response.
More complex applications may use state management frameworks such as Redux or MVI (Model-View-Intent) in order to scale well, especially with large apps.
I've used declarative state management with SwiftUI on iOS. It's pretty easy to use @State, @Binding, and @ObservedObject to handle both local and shared states. I also implemented the Combine framework for more reactive applications; thus, I could bind data and have the user interface refresh immediately when things change.
Generally, I make state management as easy and maintainable as possible and then pick a good strategy by the size and complexity of the application.
Q4. What is your process for monitoring and addressing app crashes and bugs in production?
For instance, when debugging crashes or problems at the production level, I follow the app's behavior and gather detailed crash reports with the help of technologies like Firebase Crashlytics, Sentry, or Rollbar. I get to establish readily what the root problem is using these technologies to monitor the stack trace, app status, and environment details. After that, I determine how much of a priority I need to attach to its resolution based on how the users are affected by it. Apart from this, I ensure that all logging and error-handling procedures are in place so that small defects can be found before they have an impact on the users. I test the application thoroughly in staging after applying the fix to prevent regression, then release the patch with proper release notes and a smooth deployment plan.
Q5. How can you store data in Android and iOS? Which databases and native solutions are available?
Ans. For simpler keyed-value pairs, there's SQLite for structured databases, and Room (a database abstraction layer over SQLite) for easier data handling with live data and object mapping. Besides using UserDefaults for storing key-value pairs, there is Core Data when the data model gets complex, and SQLite for relational data in iOS. Some popular ones for cross-platform application use are Realm and SQLite, as they offer efficient data handling and offline support. Another set of chosen databases includes cloud-based ones that provide scalability and real-time data syncing between iOS and Android devices, such as AWS Amplify and Firebase Firestore.
Q6. What is the best way for a server to notify an app that some new data is available?
Ans. Push notifications or instant communication protocols are the most efficient methods by which a server can update an app on new data. Even when an app is running in the background, push notifications—for example, Apple Push Notification Service (APNs) for iOS and Firebase Cloud Messaging (FCM) for Android—inform the app and remind users to view new content. WebSockets or technologies like Firebase Realtime Database and SignalR enable persistent connections. This way, the server can send data back promptly without making repeated requests for the same data at an app level. It enables real-time updates within the application. These techniques may be used one at a time or in combination, depending upon the need of the application and to be able to update the data appropriately and promptly.
Q7. How can you debug your app when it’s already released?
Ans. I use logging frameworks and monitoring tools to detect and fix issues in production when debugging a released app. The Crashlytics, Sentry, or Bugsnag crash report is recorded with stack traces and user session data for the identification of failure and its cause. Analytics tools are Mixpanel or Firebase Analytics. For added depth, I added custom logging to return nonsensitive error data back to the server. Using server-side logging, real-time crash reports, and user analytics, I could spot, prioritize, and resolve issues in a living app environment in real time.
Q8. Describe the process of publishing apps on Google Play and in Apple’s App Store. What do you need to publish an app to each store?
Ans. First, the process of the release of the applications through the Apple App Store and Google Play. In Google Play, getting the developer account and having the app bundle file ready are steps included in the procedures. The app includes a feature graphic, screenshots, an icon, and an app title with a small description. Filled-up: description, classification, content rating, and privacy statement for the app in Google Play Console. I also enabled the options for the app to go for distribution and price. Then I upload all my work and go through the specifications for a while which in most cases might take one or two hours or even more. Then I submit the app for review.
This is followed by registration with the Apple Developer Program, which marks the initiation of the process for the Apple App Store. I then prepare the iOS app (IPA file) using Xcode so that it's ready as per Apple's requirements. I also configure the App Store Connect along with all the necessary details such as the description of the app, keywords, privacy policy, and screenshots. It takes several days to finish. The process involves several testing functionalities and how it fits with the rules set in place by Apple's app store. When the developer accepts them, they publish them through the App Store.
Both shopfronts require regular maintenance and versions, which also undergo the same procedure.
Q9. How can you deal with the plethora of different screen resolutions and screen sizes out there?
Ans. Different screen sizes and resolutions require handling needs a responsive and flexible UI. I always stick to the platform-specific requirements. If developing in Android, I always use density-independent pixels (dp/sp), as well as stick to Auto Layout limitations when developing in iOS. More than this, I use weight-based distributions and relative positioning for flexible layouts. To ensure that the visuals don't lose their clarity while passing through multiple screens, I used vector graphics - such as SVGs for Android and @2x, @3x for iOS - for photographs and resources. In addition, to ensure that the app runs and looks good on various screen sizes, testing on emulators, simulators, and a variety of actual devices is just as important.
Q10. What is app sandboxing and what is it for?
Ans. A security technique by the name of application sandboxing separates the data and operations of an application from others and the operating system. Each application runs in its own "sandboxed" environment to limit direct access to memory, resources, files, and other programs in other devices, thereby protecting against unauthorized entries and leaks as well as minimizing the probable impact of malware and also the protection of sensitive user data. Importantly, because of sandboxing, which ensures that even if a dodgy application has been compromised, it cannot use those compromised capabilities to impact other applications or access important system data, it keeps user privacy and system security intact on mobile operating systems like iOS and Android.
Q11. What are the features of the Android Architecture?
Ans. Layer-based Android architecture provides a module framework for efficient development and use of apps. The key features encompassed are as follows:
- Linux Kernel: It is the system architecture, about memory, power as well as control of device drivers, and security among many other important system operations.
- Hardware Abstraction Layer (HAL): This allows Android to communicate directly with hardware; this is not based on any reference to the hardware itself, for example, cameras or sensors.
- Android Runtime Android Runtime which executes app code is also referred to as ART. It uses both Ahead-of-Time (AOT) and Just-in-Time (JIT) compilation in the management of memory and performance.
- Libraries for native C/C++: Regarding media playing capabilities, data storage features (SQLite), and graphics rendering capabilities (OpenGL),
- Java API Framework: Numerous APIs are offered with the aid of which apps are developed, like network connectivity APIs, data management APIs, and user interface designing APIs
- System Apps: These are applications such as Android's phone, contacts, and messaging that are part of the fundamental blocks and afford a useful, user-friendly interface.
This multi-layered architecture makes the app compatible across various Android devices and therefore allows for resilience, portability, and scalability.
Q12. What is Google Android SDK? Which are the tools placed in Android SDK?
Ans. Such a set of tools and frameworks like Google Android SDK (Software Development Kit) enables programmers to design, test, and release Android applications. An important core component allows one to write and debug code, simulate Android devices as well as package apps for release.
A few of the most widely used tools in the Android SDK include:
- Android Studio: It is the official IDE for Android that offers UI design and provides editing capabilities along with debugging.
- Android Emulator: It proves to be useful for testing an application on different Android versions and settings using a virtual device.
- Android Debug Bridge (ADB): It is a command-line utility that allows control and interaction with Android devices in terms of log management, debugging, and installation.
- Gradle: It is the application build automation tool that does the packaging and management of the dependency.
- SDK Manager: The control over system images, tools, updates, and SDK versions.
- Lint Tool: This gives a solution to all the possible errors that could be made by the developers, problems in performance, and the quality of the code.
In combined use, these ensure compatibility with functionality across Android devices while streamlining the app development process.
Q13. What is AIDL? Which data types are supported by AIDL?
Ans. Using a tool from Android called AIDL, or Android Interface Definition Language, an application can specify which interface it would use to communicate with other applications across processes. AIDL works by allowing services that applications may use whether or not they are in separate processes. Another example is primitive types, such as int, float, boolean, etc; and objects of String, List, Map, and parcel. AIDL supports these core data types and helps in the transfer of structured data from one process to another.
Q14. What is the life cycle of Android activity?
Ans. The lifecycle of an Android activity refers to the series of states an activity goes through from its creation to its destruction. It is important for managing resources, UI updates, and user interactions. The primary methods that represent these states are:
- onCreate(): Called when the activity is first created. This is where you initialize the activity, set the layout, and perform setup tasks like binding UI elements or initializing variables.
- onStart(): Called when the activity becomes visible to the user. The activity is about to be displayed, but it’s not yet interactive.
- onResume(): Called when the activity starts interacting with the user. It’s the most active state, and you should resume animations, listening to sensors, or any other tasks that involve user interaction.
- onPause(): Called when the system is about to resume another activity. This is where you should pause any ongoing tasks like animations or background services, and save important data (e.g., user input or app state).
- onStop(): Called when the activity is no longer visible to the user. Resources like sensors or GPS should be released here to prevent memory leaks.
- onRestart(): Called after the activity has been stopped and is about to start again. You can use this method to refresh the UI or reinitialize any necessary components.
- onDestroy(): Called when the activity is about to be destroyed. This is the place to release resources, stop background threads, and clean up before the activity is completely removed from memory.
Understanding the lifecycle ensures proper management of resources and a smooth user experience by allowing activities to handle interruptions like calls, screen rotation, or memory cleanup efficiently.
Q15. What is the difference between CompileSDKVersion and targetSDKVersion?
A. Difference between Android's `compileSdkVersion` and `targetSdkVersion`
- compileSdkVersion: Makes available all the APIs up to the version of the Android SDK used for compiling the application. It merely configures the basics of creation and building; it doesn't affect what an app is doing on some devices. New features and APIs: The stable version should be used.
- targetSdkVersion: This specifies how the system uses version-specific behaviors. This is indicated by which Android version the application has been optimized for. It is set to the latest version, thus ensuring that this provides best compatibility and system management, although the software can operate on older versions.
Q16. What is JobScheduler?
Ans. An Android API called JobScheduler enables developers to handle background operations or activities that need to be run at regular intervals of time or when a specific condition has been fulfilled—for instance, charging the device or the device being connected to the internet. This method provides more reliable and efficient execution of tasks than more conventional ones such as `AsyncTask` or `Handler`. It was initially introduced as part of Android 5.0 (Lollipop).
Q17. When to use strong, weak, and unowned references?
Ans. When to use Swift's strong, weak, and unowned references:
1. Strong References:
- Purpose: Avoid deallocation till all of your strong references are released; so the object stays in memory as long as the reference exists.
- When to apply: Apply it to objects where you want to keep and control, like a view controller that owns its view.
- Example:
```swift
var myObject = SomeClass() // Strong reference
```
2. Weak References:
- Purpose: It prevents retain cycles by allowing the object to be deallocated, if no strong references can be found.
- When to Use: When the specific item should not allow the other object from getting deallocated or if delegate properties are there, use this method.
- Example:
```swift
weak var delegate: SomeDelegate? // Weak reference
```
3. Unowned References:
- Purpose:
Expects the object to survive around it until it is referenced, but does not retain it. A crash will occur if you try to access a deallocated unowned reference.
- When to Use: Make use of this situation, like parent child relationship, where it is known that the referred object will outlive the reference.
- Example:
```swift
unowned var viewController: MyViewController // Unowned reference
```
Summary:
- Strong: Keeps an object alive; use when you need ownership.
- Weak: Allows deallocation; use to avoid retain cycles.
- Unowned: Assumes object will not be deallocated; use when reference should not outlive the object.
Q18. What is Intent in Android?
Ans. Android uses a messaging object called an intent to let an app's components or even other apps communicate with each other. The key operations the intents perform include sharing data, requesting actions, and starting broadcasts, services, or activities. Explicit intents refer to a component, specifying what that component, probably an activity in the application, should open. Implicit intents describe very general actions the system might perform, such as browsing a web page, and the system decodes which application it needs to use. For Android to send its broadcast messages, to initiate services, launch activities, or transfer data, intents are required.
Q19. What is multithreading and how do you implement it in mobile app development?
With separate threads, multithreaded operation allows multiple processes to be executed in parallel with each other so that the overall performance of an application is enhanced, coupled with a mobile development fast-to-react user interface. This way, the mobile application does not degrade into slowness by offering background tasks like data processing or file downloads without halting the primary thread user interface.
Multithreading in Android prevents the main UI thread from being blocked. Traditional methods include using threads for simple background tasks,
For more flexible concurrent task management, use ExecutorService. Whereas, Kotlin Coroutines (recommended by Kotlin) for sequential writing of asynchronous programs. The newer option, Coroutines, has taken the place of the AsyncTask technique that was used in previous versions for improved performance.
OperationQueue and Grand Central Dispatch (GCD) are widely used in iOS, but while OperationQueue gives you additional control over concurrent activities (letting you defer or perform operations based on dependencies), GCD lets you dispatch tasks asynchronously to different queues-one of which is the main queue and others background queues.
Important Points Maintain all the updates on the user interface in the main thread. Avoid race situations by controlling synchronization. Any mobile application that runs with several processes should employ multithreading in order to maximize responsiveness.
Q20. How do you ensure app's security?
There are a number of steps that ensure an application is protected. First, I encrypt sensitive data at rest and in motion. For instance, to have safe communication, I use TLS encryption followed by AES encryption used in the storage of data. Powerful authentication techniques involving OAuth, biometrics, and multi-factor authentication come into play when performing user authentication. I also follow secure coding standards, for instance, code obfuscation, and exclusion of hard-coded private data, such as API keys and passwords. I also use HTTPS-enabled secure API endpoints using the right authorization tokens in place. Such vulnerabilities get identified and remedied through regular security testing and vulnerability assessments. For example, by using Firebase Crashlytics, among other technologies, I watch for questionable activity and consequently update in a timely manner.
Q21. What is Android's ViewModel and how does it work?
Ans. The Android Architecture Components include a ViewModel, which is used to store and handle UI-related data in a lifecycle-aware manner. It improves performance and user experience by enabling data to withstand configuration changes, such as screen rotations, minimizing memory leaks, and avoiding needless data reloads.
Q22. What are the advantages of dependency injection when developing mobile apps?
Ans. By supplying object dependencies from outside sources instead of generating them inside, dependency injection (DI) makes managing them easier. It increases modularity and testability, which facilitates implementation changes and is particularly helpful for large apps. Android frequently uses well-known libraries like Hilt, Koin, and Dagger for DI.
Q23. What is the difference between MVC and MVVM in mobile app development?
Ans. Code can be structured using design patterns like MVVM (Model-View-View Model) and MVC (Model-View-Controller). Though MVC keeps data, UI, and logic separate, it gets messy when it is big. With MVVM, which is recommended in Android, separates business logic from UI code by adding a ViewModel to manage data related to UI which is easier to test and maintain.
Q24. What is the difference between MutableLiveData and LiveData?
Ans. LiveData is an Android class for holding and observing data in a lifecycle-aware way. It automatically resynchronizes and updates user interface elements and reacts to changes in the underlying data. Even though LiveData is immutable, hence read-only, its subclass supports data binding and therefore allows the data to be changed.
Q25. How does Swift handle error management?
Ans: Swift handles errors in a clean fashion, by using the `try`, `catch`, and `throw` keywords. Specifying the `Error` protocol defines what constitutes an error, which then gets thrown via `throw`. The outcome is that the `do-catch` block cleans up these errors elegantly, leaving apps more reliable and allowing issues to be addressed without crashing apps.
Q26. What are Kotlin's higher-order functions and what do they do?
Ans. Functions in Kotlin that return other functions as their results or that accept functions as parameters are higher-order functions. It is, however, the support to which back-end operations like filtering and mapping occur through the feature of functional programming. These are vital to writing cleaner, better maintainable code in the development process of an Android app.
Q27. What does CoreData do in iOS development?
Ans. CoreData: This means a good iOS framework for managing and saving data in applications. It is also a persistence framework and an object graph, meaning it allows developers to store, retrieve, and change app data in an effective way. It's ideal for programs that will require having some information stored locally because it supports complex data structures and relationships.
Q28. How does Kotlin handle null safety?
Ans. The separation of nullable and non-nullable types in Kotlin's type system prevents null pointer exceptions and provides null safety. Safer code is enforced by nullable types in which nulls are explicitly allowed, such as with `String?`, while nulls are not possible for non-nullable types, such as `String`. Runtime crashes are reduced with the operators `?.` and `?:`, which simplify the handling of nulls.
Q29. What is Android Broadcast Receiver?
Ans. In Android, a broadcast receiver listens for broadcast messages uniquely for an application or the whole system such as low battery, connectivity, etc. Because apps can react to changes even when they are not running in the background, they allow programs to be controlled based on system events or custom intents, saving resources.
Q30. What is the ProGuard and how does it help android apps?
Ans. A tool called ProGuard compresses APKs and obscures them to be harder to read by compressing, obfuscating, and shrinking the codes of Android applications. It obfuscates classes, fields, and methods, removes unused code, and increases security and performance. It is particularly useful for protecting important business logic.
Conclusion
In conclusion, you can increase the chances of clearing your mobile application developer interview if you prepare with the key ideas and sophisticated strategies covered in this post. Knowing these guidelines might actually aid you in writing better, more effective, and more user-friendly applications, besides increasing your self-confidence during interviews. Now that you are well-equipped to understand these subjects, you're finally ready to showcase your potential and make them remember you as a proficient and knowledgeable developer.
FAQs
Q. What's the best way to discuss past failures or challenges in a Mobile App Developer interview?
Ans. Discuss earlier mistakes: Focus on actions taken in finding the problem and correcting it, as well as what you have learned from that experience. Emphasize that this taught you something and made your skills more improved in solving future problems.
Q. How can I effectively showcase problem-solving skills in a Mobile App Developer interview?
Ans. Show some problem-solving skills by narrating specific challenges you faced while presenting technological concepts, your approach to dealing with them, and the positive outcomes you achieved. Use the STAR model example: Situation, Task, Action, Result.
Q. What blunders can one avoid at the time of presentation of technological concepts during an interview?
Ans. Explain concepts simply and clearly, avoiding technical jargon whenever possible. Attempt to make your understanding more concrete by relating technical concepts to applications or past project experience.
Q. How important is it to know Android and iOS as well?
Ans. While specialization in either iOS or Android is valuable, having familiarity with both can make you more versatile and adaptable, especially in environments where cross-platform skills are valued. It also shows your willingness to learn and adapt to various mobile ecosystems.