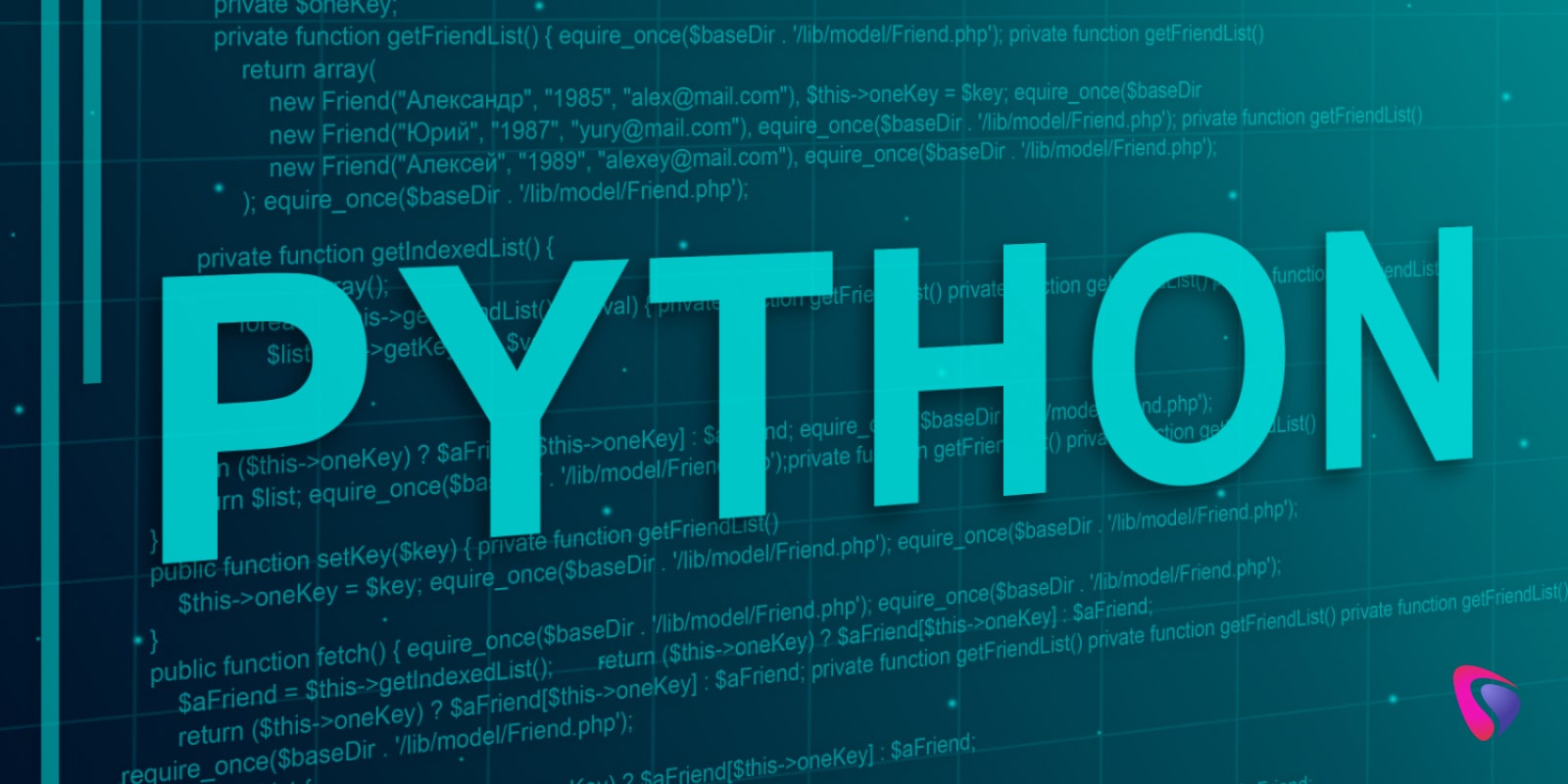
Even the most skilled and experienced programmers might miss some of the most potent features of Python, given the language's well-known simplicity and adaptability. You will be able to write neater, more effective, and more elegant code by mastering these ingenious techniques. Routine chores will become streamlined and intricate operations smoothed out as you master these Python tips to improve your coding skills and increase output. Regardless of your level of experience, these pointers will make learning Python easier and more pleasurable. Let's investigate the realm of mastering Python!
Why Python is trending?
With the ease of use, flexibility, and immense ecosystem, Python is one of the most widely used programming languages in cutting-edge technologies. The TIOBE Index has ranked Python as the leading programming language for years. Python is one of the most sought-after and popular languages and has over 44% of its users, according to Stack Overflow's Developer Survey 2023 report.
According to Anaconda, 70% of data scientists use Python for activities like machine learning and data analysis, proving its adoption across a variety of industries. Moreover, millions of repositories rank Python in the top three languages in services like GitHub, with extensive usage in web development, automation, artificial intelligence, and more. Because of its strong community and constantly expanded library, Python has become the choice for developers in various fields. Hire dedicated python developers for your next project.
Amazing Python Tricks That Will Instantly Improve Your Code
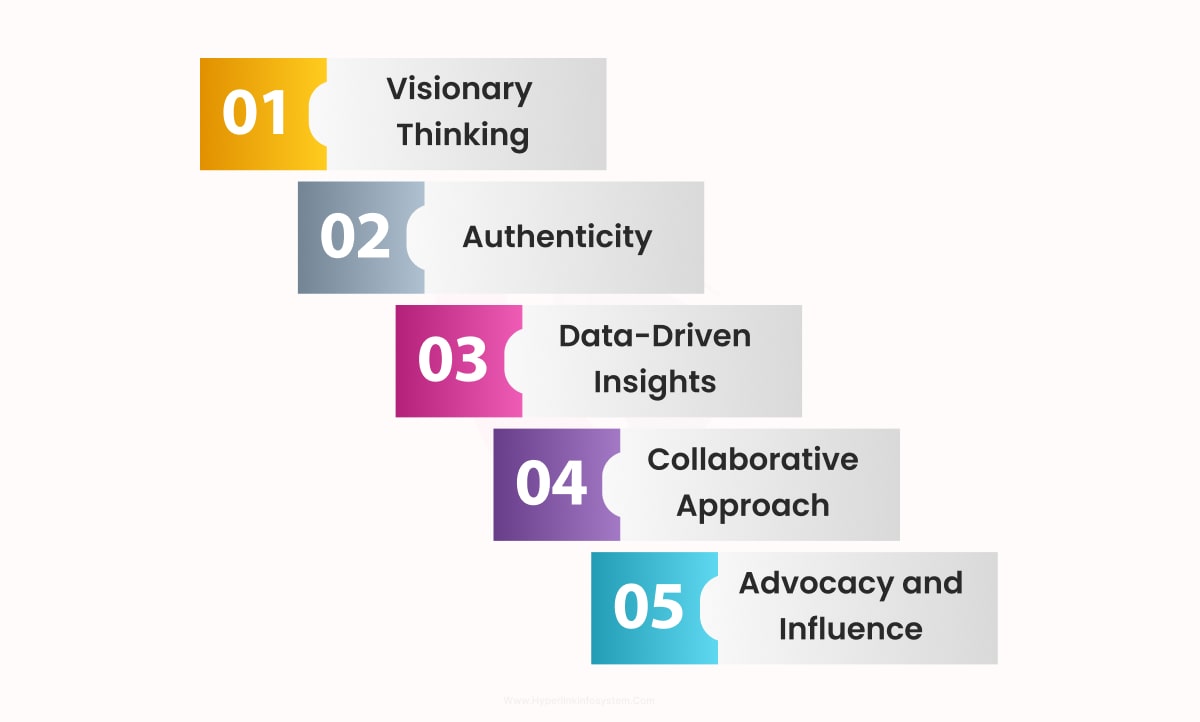
Slicing
Slicing is used to get access to characters from any string, list, or tuple. You can access characters using the slicing method by specifying their indexes. The slicing feature is used to make the code more cleaner, more concise, and more readable. By using this function, you can access the characters of a string without actually modifying the original data.
Code:
x = "This is my first python code"
print = (x[2:9])
Output:
"is is my"
In this code, by using the slicing method, python will give you characters from index 2 to index 9.
Merging Dictionaries
By using this method, programmers can merge their dictionaries. In Python 3.9 and other above versions, you can merge dictionaries using the "|" - OR operator.
Code:
x = {"L": 1, "M": 2}
y = {"N" : 3, "O": 4}
x_and_y = x | y
print (x_and_y)
Output:
{"L":1, "M":2, "N":3, "O":4}
The 'not' Operator
A not operator in Python is one of the logical operators used to negate a boolean expression. It is one of the simplest and most intuitive operators in Python's logic that helps to evaluate conditions or expressions by inverting the truth value. In simpler terms, it converts a True expression to a False, and a False expression to True.
Code:
a = []
print(not a)
Output:
True
The Print Functions 'end' Parameter
If you want to control your output to some degree then this function will help you do it. By default, Python will print each item with a new line. By using the "end" parameter, you can control what is displayed in the print statement.
Code:
x = ["Hi", "There", "Hello", "Mate"]
for language in x;
print(language, end=" ")
Output:
Hi There Hello Mate
Append Function
The append() method in Python is used to add an item to the end of a list. It modifies the original list by appending the given element.
Code:
# initialize list
a = [1, 2, 3]
# append a value
a.append(4)
print(a)
Output:
[1, 2, 3, 4]
Generators
Generators are a special type of function in Python that allows us to create an iterator in a more memory-efficient way. Instead of returning a single value, generators "yield" values one at a time using the yield keyword.
Code:
def simple_generator():
yield 1
yield 2
yield 3
gen = simple_generator()
# print values
for value in gen:
print(value)
Output:
1
2
3
Lambda Functions
It allows us to create a one-line, small, anonymous function. They behave as normal functions only except lambda functions don't have names.
Code:
square_lambda = lambda x:x**2
print(f"Lambda function: {square_lambda(4)}")
Output:
Lambda function: 16
Ternary Operator
The ternary operator works as a conditional expression among names. The ternary operator provides results based on whether the condition is true or not.
Code:
condition = True
name = "John" if condition else "Doe"
print(name)
Output:
John
Remove Duplicates From Lists
You can remove duplicates from lists using the 'set' method. By using this method, you can improve app performance.
Code:
x = [7, 7, 5, 5, 5, 1, 2, 3, 2, 5, 7]
print(list(set(x)))
Output:
[7, 5, 1, 2, 3]
The Print Functions 'sep' Parameter
As we discussed above, programmers can control their output. With the 'sep' parameter, you can control what to show between the space of each item.
Code:
day: "14"
month: "12"
year: "2006"
print(day, month, year)
print(day, month, year, sep= "")
print(day, month, year, sep= ".")
Output:
14 12 2006
14122006
14.12.2006
Matching Regex
You can search defined patterns or text using Regular expressions.
Code:
import re
text = "A white dog is sitting with other dogs in the dog park."
pattern = r"dog"
matches = re.findall(pattern, text)
print(f" Occurences of 'dog' :{matches}")
Output:
Occurrences of 'dog': ['dog', 'dog', 'dog']
Regex Pipe
Regular expressions use '|' operator which represents OR so that you can find out and match any of the given patterns with the given string or text.
Code:
import re
healthy = re.compile(r''super(fruit|vegetables|food)")
h1 = healthy.search("Mango is a superfruit.")
h2 = healthy.search("Pumpkins are not considered as supervegetables.")
h3 = healthy.search("Superfood boosts a healthy life.")
print(h1group.())
print(h2group.())
print(h3group.())
Output:
superfruit
supervegetables
superfood
The 'SwapCase' Method
This method allows to change the case of the text in the program. You can change lower case into upper case and upper case into lower case as per your requirements.
Code:
string = "HI, TherE! I Am A RoBOt"
print(string.swapcase())
Output:
hi, tHERe! i aM a rOboT
The 'isalnum' Method
We are creating a program that requires users to enter a password. The password must have both letters and numbers. To check this, we can use the `isalnum()` method on the string. This method confirms that all characters are either letters (A-Z, a-z) or numbers (0-9). If the string has any spaces or symbols (like !, #, %, $, &, ?), the method will return False.
Code:
password: "ABCabc123"
print(password.isalnum())
Output:
True
Exception Handling
Exception handling is the best way to manage run-time errors. By using exception handling, you can ensure that the program deals with issues without crashing the system.
Code:
def get_ratio(x:int, y:int) -> int:
try:
ratio = x/y
except ZeroDivisionError:
y = y + 1
ratio = x/y
return ratio
print(get_ratio(x=500, y=0))
Output:
500.0
Identifying the Differences in the Lists
In Python, the differences between two lists can be easily ascertained with sets and various operations on them, such as simple subtraction, symmetric difference operator (^), or symmetric_difference() method.
Code:
list_x = [1, 3, 5, 7, 8]
list_y = [1, 2, 3, 4, 5, 6, 7, 8, 9]
output_1 = list(set(list_y) - set(list_x))
output_2 = list(set(list_x) ^ set(list_y))
output_3 = list(set(list_x).symmetric_difference(set(list_y)))
print(f"Solution 1: {output_1}")
print(f"Solution 2: {output_2}")
print(f"Solution 3: {output_3}")
Output:
Solution 1: [9, 2, 4, 6]
Solution 2: [2, 4, 6, 9]
Solution 3: [2, 4, 6, 9]
List Comprehension
List comprehension is a concise way to create lists in Python. You can create better lists using comprehension than typical loop methods. With list comprehension, you can apply conditions or iterations to each variable in a single line.
Code:
even_numbers = [x for x in range(20) if x % 2 == 0 and x != 0]
print(even_numbers)
Output:
[2, 4, 6, 8, 10, 12, 14, 16, 18]
Aliasing
Aliasing is a concept where two different identifiers reference the same object.
Code:
x = [1, 2, 3, 4 ,5]
y = x
# Change the 4th index in y
y[4] = 7
print(id(x))
print(id(y))
print(x)
Output:
1, 2, 3, 7, 5
Args and Kwargs
This *args parameter will allow a function to receive a variable number of arguments, although it's only usable in non-keyworded mode. However, the **kwargs parameter can be used for allowing an arbitrary number of keyworded parameters to the function.
Code:
def some_function(*arg, **kwargs):
print(f"Args: {args}")
print(f"Kwargs: {Kwargs}")
Output:
Args: (1, 2, 3)
Kwargs: {'a' : 4, 'b' : 5, 'c' : 6}
F-strings
Occasionally, we may need to format a string object; Python 3.6 introduced a cool feature called f-strings to simplify this process. It helps to understand how strings were formatted before the new release to appreciate the new method better.
Code:
first_name = "Henry"
age = 25
print(f"Hi, I'm {first_name} and I'm {age} years old!")
Output:
Hi, I'm Henry and I'm 25 years old!
Underscore Visual
In Python REPL (Read-Eval-Print Loop), the underscore _ holds the result of the last executed expression. It is useful for quick calculations or referencing the previous result.
Code:
10 + 20
_ * 2
Output:
30
60
Standalone Underscore
The standalone underscore _ is often used as a throwaway variable when the value is not needed. It is helpful in loops, unpacking, or capturing unused return values, making the code cleaner and easier to understand.
Code:
for _ in range(3):
print("Hello!")
Output:
Hello!
Hello!
Hello!
__name__ == "__main__"
This conditional block determines whether or not a Python script is run directly versus being imported into some other program as a module. While when run it causes the block to execute if run independently, otherwise it's ignored, thus providing reusable code without unwanted execution.
Code:
if __name__ == "__main__":
print("This script is run directly!")
Output:
This script is run directly!
Sorting with Key
Python's sorted() and .sort() allow sorting with a custom key function. This helps in sorting complex objects or using non-default criteria such as length or a specific attribute.
Code:
names = ["Harry", "Zayn", "Tom"]
sorted_names = sorted(names, key=len)
print(sorted_names)
Output:
['Tom', 'Zayn', 'Harry']
Enumerate
The enumerate() function adds a counter to an iterable, making it easy to track indices while iterating. This is particularly useful for loop counters in Python.
Code:
for index, value in enumerate(['a', 'b', 'c']):
print(index, value)
Output:
0 a
1 b
2 c
Checking for Substring
Python’s in operator lets you easily check if a string contains a specific substring. It’s a concise and readable way to handle substring checks.
Code:
text = "Python is amazing!"
print("Python" in text)
Output:
True
Flatten a List of Lists
A list of lists can be flattened using list comprehensions or itertools.chain(), making it a one-dimensional list. This simplifies further data processing.
Code:
nested = [[12, 21], [35, 45], [5]]
flat = [item for sublist in nested for item in sublist]
print(flat)
Output:
[12, 21, 35, 45, 5]
Reversed Iteration
The reversed() function iterates through a sequence in reverse order without altering the original sequence. It’s an efficient way to handle reverse iteration.
Code:
for char in reversed("Python"):
print(char, end="")
Output:
nohtyP
String Join
The join() method efficiently concatenates a list of strings into a single string, separating them with a specified delimiter, often saving time and memory.
Code:
words = ["Python", "is", "fun"]
sentence = " ".join(words)
print(sentence)
Output:
Python is fun
The Ellipsis
The ... (ellipsis) is a placeholder often used in typing hints, slicing, or marking sections of incomplete code in Python.
Code:
def future_function():
... # TODO: Implement this later
print(future_function)
Output:
<function future_function at 0x...>
Unpacking
Python’s unpacking feature lets you assign elements of an iterable to variables in one step. It works seamlessly for lists, tuples, and more, reducing redundancy.
Code:
a, b, c = [1, 2, 3]
print(a, b, c)
Output:
1 2 3
Conclusion
Python's versatility and ease of use for developers have completely transformed the programming environment. It is extensively used in many domains, including data science, automation, artificial intelligence, and web development, and it fosters breakthroughs in various businesses. The large library, robust community, and ability to handle a variety of jobs make Python a vital tool in contemporary software development.
Working with experienced developers is a must to fully utilise the power of Python. Your projects will be executed efficiently, scale well, and be innovative if you hire Python developers or hire Python development company. Whether you are a startup or an established business, having the right Python knowledge will help you expand and realize your ideas. To ensure a successful solution that is future-proof, go with Python development! Contact us today!
FAQs
Q- What are some ways to improve my Python code?
Ans. Make use of Python libraries, write modular and reusable code, follow PEP 8 standards, and use linters like flake8 to find problems. Regularly test and refactor code for better maintainability.
Q- How can I make my Python run faster?
Ans. Use effective algorithms, use libraries like NumPy or Cython, and use functools.lru_cache to build caching in order to optimise code. Use external tools like PyPy or multiprocessing for complex calculations.
Q- How to code Python like a pro?
Ans. We need to learn basic Python, experiment with more complex methods like decorators and context managers, and become familiar with the libraries and frameworks that are pertinent in our field. Clear, understandable, and effective code will be crucial, as well as keeping up with new Python capabilities.
Q- How much faster is Python 3.12?
Indeed, CPython interpreter optimisations and memory usage in Python 3.12 significantly improve speed. Benchmarks against Python 3.11 show increases in the speed of many processes of as much as 5%.